Redux란 ?
Redux 는 상태관리 라이브러리 라고 합니다.
상태관리 라이브러리 라고 하면 처음 접하는 분들에게는 어렵게 느껴질 수 있다고 생각합니다. 그래서 저는 그냥 간단하게 Hooks 함수 중에 useState를 제약없이 (?) 사용하게 해주는 라이브러리라고 생각합니다.
실제로 Redux를 사용하는 이유 중에 보통 React 에서는 props를 통해 값을 넘겨주게 되는데 자식 컴포넌트가 100개 아니 수억개가 존재하면 계속해서 props를 넘겨줘야하기 때문에 코드가 엄청나게 길어집니다.
하지만 Redux를 사용하면 모든 컴포넌트가 공유하는 상태값을 저장할 수 있으니 편하게 된다고합니다.
설치하기
yarn add redux react-redux
or
npm install redux react-redux
해당 프로젝트에 설치해줍니다.
리덕스에서는 필수로 알아야하는 몇가지가 존재합니다.
1.store
store는 컴포넌트와 별개로 존재하며, 상태값을 저장하는 공간 이라고 생각하면됩니다.
2.action
action은 스토어에 운반할 데이터를 의미합니다.
3.reducer
reducer는 action만 있다고 데이터를 운반하는 것이 아니고 reducer를 따라야 합니다.
action을 reducer에 전달한 후 store를 최신화 해주는 겁니다.
살짝 switch 문이랑 비슷하다고 생각합니다.
실습
index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import { Provider } from 'react-redux';
const store = createStore()
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<Provider store={store}>
<React.StrictMode>
<App />
</React.StrictMode>
</Provider>
);
reportWebVitals();
createStore는 store를 생성해주는 함수.
Provider는 store를 사용할 컴포넌트를 감싸기 위해 필요한 컴포넌트
라고 생각해주시면됩니다.
cart.js
// Actions
export const ADD = "ADD"
export const add = (id,name,price,amount,src,category) => {
return {
type: ADD,
id,
name,
price,
amount,
src,
category
}
}
// 초기값 설정
const initialState = {
list : [
{
id : '',
name : '',
price : '',
amount : '',
src : '',
category : ''
}
]
};
export default function counter(state = initialState, action) {
switch (action.type) {
case ADD:
return {
list : [...state.list ,
{
id : action.id,
name : action.name,
price : action.price,
amount : action.amount,
src : action.amount,
category : action.category
}
]
};
default:
return state;
}
}
src에 modules라는 폴더를 생성해 준 후 다음과 같이 '액션함수' 를 만들어 주고,
초기값을 설정해준 후 리듀서 함수를 작성해줍니다.
Test.js
저는 간단하게 구현 하기만 하기위해서 Test.js 라고 테스트용 파일을 생성했습니다.
import React from "react";
import { useDispatch, useSelector } from "react-redux";
import {add} from "../modules/cart"
function Test(){
const dispatch = useDispatch()
const list = useSelector((state) => state.cart.list)
return(
<div>
<div>
<button onClick={()=>{dispatch
(add('id','name','price','amount','src','categoey'))}}>
add</button>
<div>
{list.map((lst) => {
return(
<div>
<h2> id : {lst.id} </h2>
<h2> name : {lst.name} </h2>
<h2> price : {lst.price} </h2>
<h2> amount : {lst.amount} </h2>
<h2> src : {lst.src} </h2>
<h2> category : {lst.category} </h2>
</div>
)
})}
</div>
</div>
)
}
export default Test
이렇게 코드를 작성해줍니다.
useSelector는 store의 값을 불러오는 Hook
useDispatch는 action을 전달해주는 Hook 정도로 이해하시면 될거같습니다.
rootRecucer.js
import { combineReducers } from "redux";
import cart from "./cart";
// import한 리듀서 이름을 그대로 사용하는 경우
export default combineReducers({
cart
});
이 파일은 리듀서 파일들이 많아 질때 한번에 합쳐서 리듀서들을 통합(?) 해주게 하는 파일입니다.
모든 리듀서파일들을 import 해준후 combineReducers에 넣어주면 됩니다.
index.js
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import { BrowserRouter } from 'react-router-dom';
import { createStore } from 'redux';
import { Provider } from 'react-redux';
import rootReducer from "./modules";
let store = createStore(rootReducer);
ReactDOM.render(
<React.StrictMode>
<BrowserRouter>
<Provider store={store}>
<App />
</Provider>
</BrowserRouter>
</React.StrictMode>,
document.getElementById('root')
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
마지막으로 createStore에 rootReducer을 넣어주면서 완성 하면 됩니다!
감사합니다.
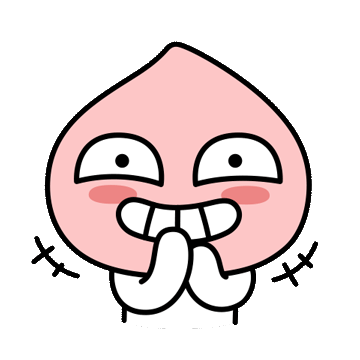
'React' 카테고리의 다른 글
React-toastify 사용하기 (0) | 2022.11.13 |
---|---|
React 에서 onChange 함수 이용하기! (0) | 2022.10.23 |
React 에서 Fontawesome 사용하기 (0) | 2022.09.08 |
React 에서 2가지 이상 import 하기 (0) | 2022.09.08 |
React 에서 axios 사용하기 ! (0) | 2022.09.05 |